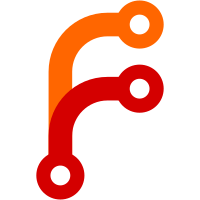
Otherwise it won't actually test the change of state.message and it would fail to check if the user missed changing state.message This happened to me as I had a catch-all line in `match`
73 lines
1.7 KiB
Rust
73 lines
1.7 KiB
Rust
// enums3.rs
|
|
//
|
|
// Address all the TODOs to make the tests pass!
|
|
//
|
|
// Execute `rustlings hint enums3` or use the `hint` watch subcommand for a
|
|
// hint.
|
|
|
|
// I AM NOT DONE
|
|
|
|
enum Message {
|
|
// TODO: implement the message variant types based on their usage below
|
|
}
|
|
|
|
struct Point {
|
|
x: u8,
|
|
y: u8,
|
|
}
|
|
|
|
struct State {
|
|
color: (u8, u8, u8),
|
|
position: Point,
|
|
quit: bool,
|
|
message: String
|
|
}
|
|
|
|
impl State {
|
|
fn change_color(&mut self, color: (u8, u8, u8)) {
|
|
self.color = color;
|
|
}
|
|
|
|
fn quit(&mut self) {
|
|
self.quit = true;
|
|
}
|
|
|
|
fn echo(&mut self, s: String) { self.message = s }
|
|
|
|
fn move_position(&mut self, p: Point) {
|
|
self.position = p;
|
|
}
|
|
|
|
fn process(&mut self, message: Message) {
|
|
// TODO: create a match expression to process the different message
|
|
// variants
|
|
// Remember: When passing a tuple as a function argument, you'll need
|
|
// extra parentheses: fn function((t, u, p, l, e))
|
|
}
|
|
}
|
|
|
|
#[cfg(test)]
|
|
mod tests {
|
|
use super::*;
|
|
|
|
#[test]
|
|
fn test_match_message_call() {
|
|
let mut state = State {
|
|
quit: false,
|
|
position: Point { x: 0, y: 0 },
|
|
color: (0, 0, 0),
|
|
message: "hello world".to_string(),
|
|
};
|
|
state.process(Message::ChangeColor(255, 0, 255));
|
|
state.process(Message::Echo(String::from("Hello world!")));
|
|
state.process(Message::Move(Point { x: 10, y: 15 }));
|
|
state.process(Message::Quit);
|
|
|
|
assert_eq!(state.color, (255, 0, 255));
|
|
assert_eq!(state.position.x, 10);
|
|
assert_eq!(state.position.y, 15);
|
|
assert_eq!(state.quit, true);
|
|
assert_eq!(state.message, "Hello world!");
|
|
}
|
|
}
|